Quick AI Chatbot Actions with DigitalOcean Functions and Copilot Studio
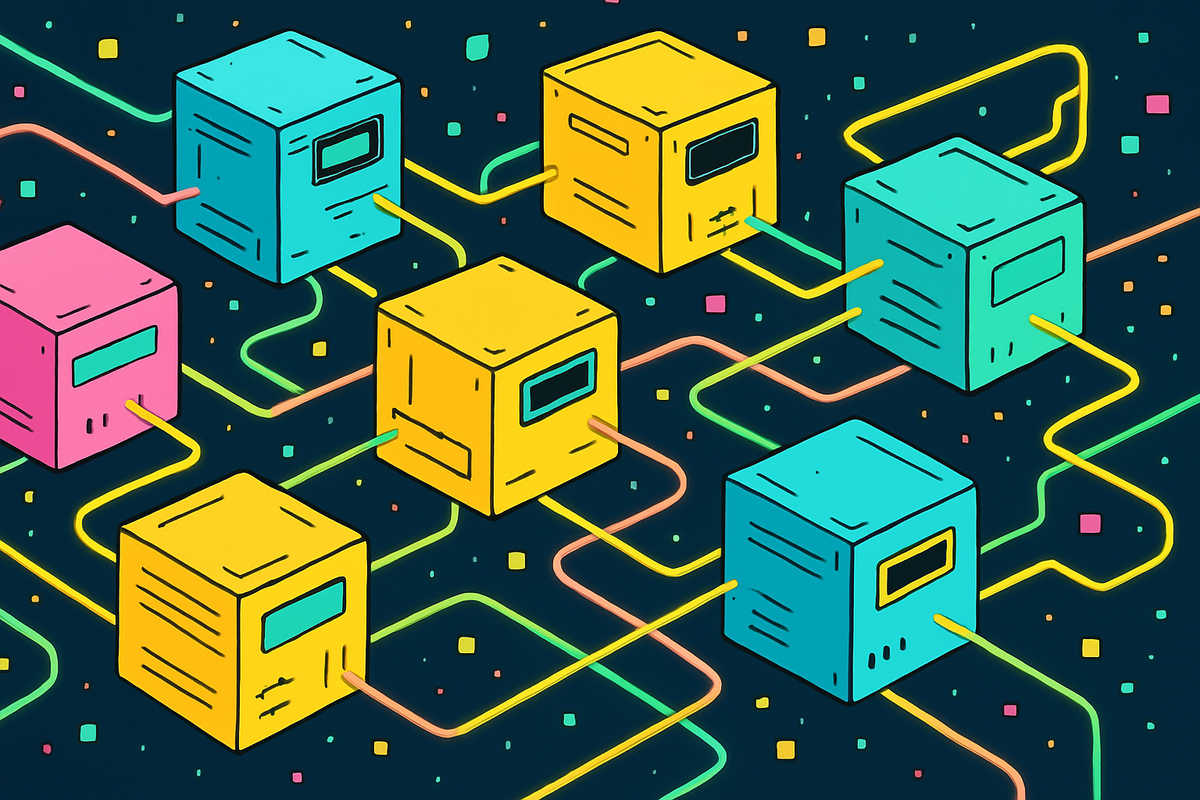
AI chatbots are powerful on their own, but they often need a little help to perform specific tasks. For example, imagine your chatbot giving instant price quotes or checking the weather – these tasks require some computation or external data. Instead of overloading your chatbot, you can delegate such work to serverless functions. DigitalOcean Functions is a great fit for this, because it's serverless (no servers for you to manage) and very easy to use.
You can deploy a small Python function on DigitalOcean in minutes and call it from your chatbot whenever needed. Plus, DigitalOcean Functions has a generous free tier (around 90,000 GB-seconds of compute per month) that makes it easy to get started without worrying about cost.
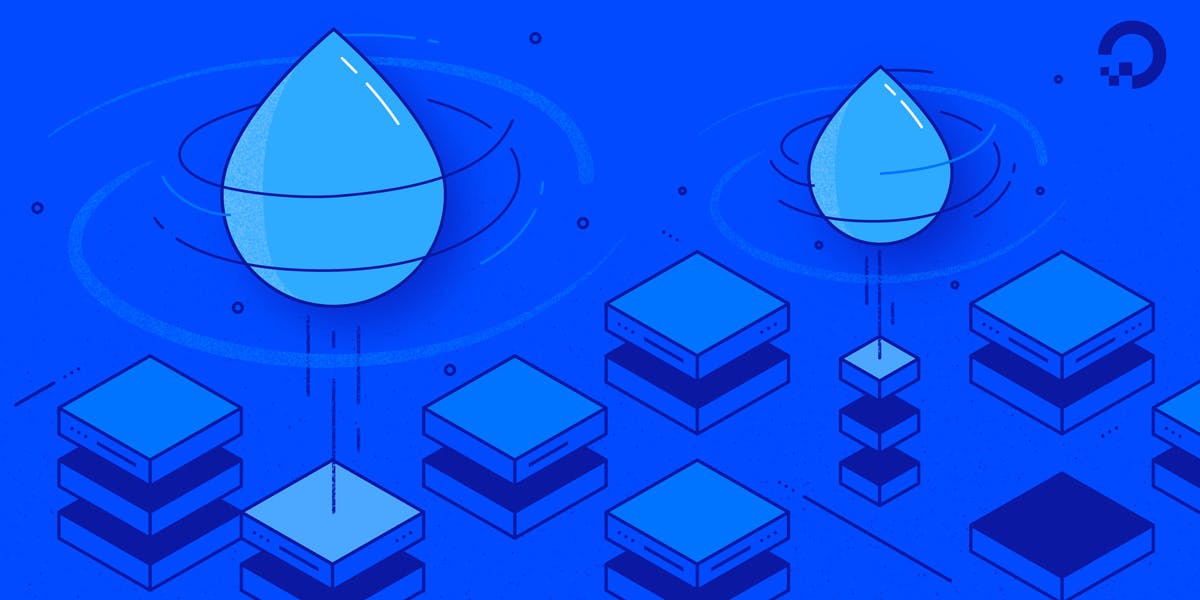
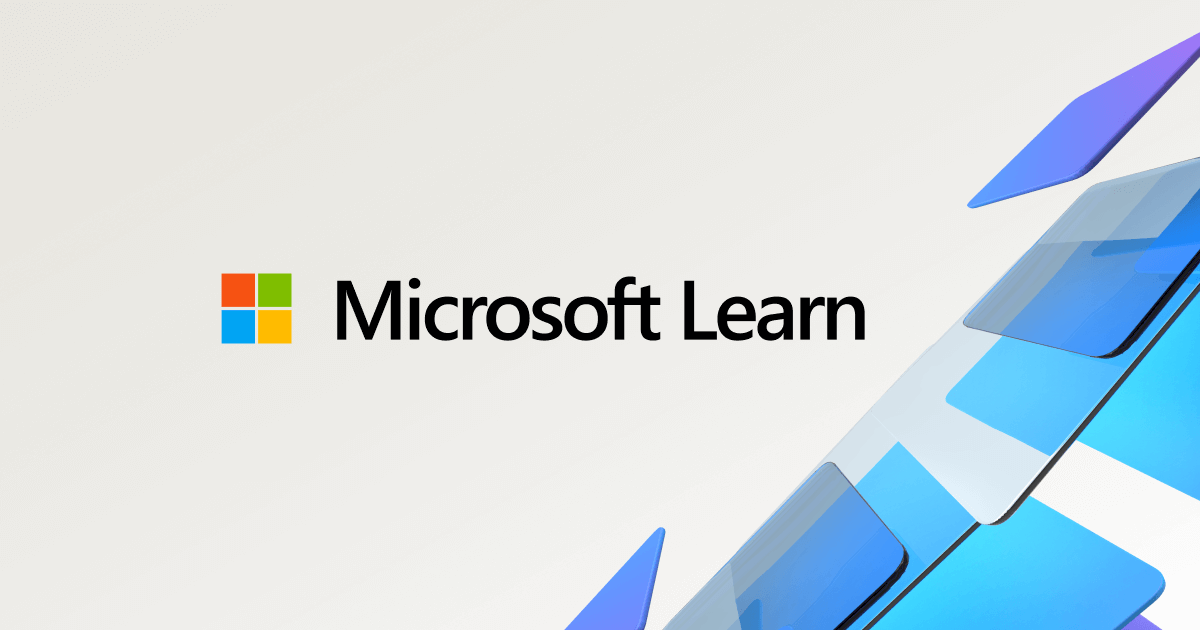
In this post, we'll walk through creating a simple Python function (a cost calculator for instant price quotes), deploying it to DigitalOcean, and integrating it as an AI action in Microsoft Copilot Studio. By the end, your AI agent will be able to call this function to answer user questions with real-time calculations.
Why Use DigitalOcean Functions for Chatbots?
DigitalOcean Functions is a Function-as-a-Service (FaaS) platform that lets you run code on-demand without managing infrastructure. Here are a few reasons it's ideal for chatbot actions:
- No Infrastructure Fuss: You write code, and DigitalOcean runs it for you. No servers to maintain or scale — it’s all handled behind the scenes.
- Fast Deployment: You can push new functions or updates in seconds, enabling rapid iteration and faster time to market for new bot features.
- Scalable & Efficient: Functions automatically scale to handle incoming requests and you pay only for what you use (down to milliseconds of execution time). There’s even a free tier to handle a good number of requests before any costs incur.
- Flexible with Python: DigitalOcean Functions supports Python (along with Node.js, Go, etc.), so you can write your action logic in a language you're comfortable with. Need extra libraries? Functions can include dependencies, or use built-in ones like
requests
for API calls. - Great for Chatbot Logic: Serverless functions make perfect microservices for chatbot backends. They can handle anything from calculations to database lookups. In fact, DigitalOcean’s own GenAI chatbot architecture suggests using Functions to add custom business logic and flexibility behind the scenes.
- Easy Integration: Once deployed, a function gets its own HTTPS endpoint. Copilot Studio (and other chatbot frameworks) can call that endpoint as a REST API, making integration straightforward.
Example Scenario: Instant Price Quote Calculator
To make this concrete, let's build a simple cost calculator function. Imagine a user asks our chatbot: "How much would 5 items cost at £3 each?" We want the bot to instantly calculate and respond with the total (which should be £15 in this case). We'll create a Python function that takes a price and quantity, multiplies them, and returns the result. This logic will live on DigitalOcean Functions, and our Copilot Studio agent will call it as needed.
It's worth noting that this is a simple example. In a scenario where the pricing calculations are more complex this method of outsourcing the math to a proper function is far superior than using the built in math abilities of LLMs.
Python Function Code – Cost Calculator
We'll write a minimal Python function for the cost calculation. DigitalOcean Functions looks for a main
function as the entry point when your function is invoked. Here's the code:
# cost_calculator.py
import json
def calculate_total_cost(quantity, price):
"""
Calculates the total cost based on quantity and price.
Ensures inputs are valid numeric values.
"""
try:
quantity = float(quantity)
price = float(price)
except ValueError:
raise ValueError("Quantity and price must be numeric values.")
if quantity <= 0 or price < 0:
raise ValueError("Quantity must be greater than zero and price must be non-negative.")
return round(quantity * price, 2) # Ensure output is a properly rounded float
def main(request):
"""DigitalOcean Functions handler. Expects a JSON payload with 'quantity' and 'price'."""
if not isinstance(request, dict):
return {
"statusCode": 400,
"headers": {"Content-Type": "application/json"},
"body": json.dumps({"error": "Invalid request format. Expected a JSON object."})
}
quantity = request.get("quantity")
price = request.get("price")
if quantity is None or price is None:
return {
"statusCode": 400,
"headers": {"Content-Type": "application/json"},
"body": json.dumps({"error": "Missing 'quantity' or 'price' parameter"})
}
try:
total_cost = calculate_total_cost(quantity, price)
except Exception as e:
return {
"statusCode": 500,
"headers": {"Content-Type": "application/json"},
"body": json.dumps({"error": "Error calculating total cost", "message": str(e)})
}
return {
"statusCode": 200,
"headers": {"Content-Type": "application/json"},
"body": json.dumps({"total_cost": total_cost})
}
Let's break down what's happening:
- We define
main(request)
– DigitalOcean calls this function when the endpoint is hit. Therequest
parameter is a Python dictionary containing any inputs passed in (as JSON payload). - The function extracts
quantity
andprice
from the request. If either is missing, it returns a 400 error instead of defaulting to zero, ensuring data integrity. - It then validates the inputs:Ensures
quantity
andprice
are numeric.Ensuresquantity
is greater than zero andprice
is non-negative.If any validation fails, a 400 or 500 error response is returned with a clear error message. - If all inputs are valid, it calculates
total_cost
asquantity * price
, rounding the result to two decimal places for clean pricing. - Finally, it returns a structured JSON response:On success (
200
) →{ "total_cost": 17.50 }
On error (400
or500
) →{ "error": "Invalid input format", "message": "Quantity must be greater than zero and price must be non-negative." }
Deploying the Function to DigitalOcean Functions
Now that our Python code is ready, let's deploy it on DigitalOcean's serverless platform. Deployment will give us a live URL endpoint we can invoke from our chatbot. Follow these steps to get your function online:
- Set up DigitalOcean Functions: Ensure you have a DigitalOcean account and install the DigitalOcean command-line tool doctl. Log in via
doctl
and connect to a Functions namespace (an isolated environment for your functions) by runningdoctl serverless connect
. This will configure your CLI to use your functions namespace. (If you don't have a namespace yet, the connect command will guide you to create one.) - Initialise a project: Use
doctl
to scaffold a new functions project. For example:doctl serverless init --language python my-chatbot-functions
. This creates a directorymy-chatbot-functions
with a sample project structure for Python. It includes aproject.yml
config and apackages/
folder for your functions. - Add your function code: In the project directory, find the sample function (e.g.
packages/sample/hello/
). You can replace the sample code with our cost_calculator code, or create a new folder underpackages
for it. Ensure the Python file (e.g.cost_calculator.py
) contains themain
function as shown above. You need to update theproject.yml
config file found in the root of the folder if you choose to create a separate package folder. - Deploy to DigitalOcean: From the project root, run:
doctl serverless deploy my-chatbot-functions
. This command bundles and uploads your functions to DigitalOcean. On success, you'll see output listing the deployed function.
You can rundoctl serverless functions get sample/hello --url
to retrieve the function’s endpoint URL. Copy this URL – we'll need it for integration. - Test the function (optional): It's a good idea to test your function. You can invoke it right from the CLI:
doctl serverless functions invoke sample/hello -p quantity:5 -p price:3
. This passesquantity=5
andprice=3
as parameters. You should get a JSON response with the total cost. (You can also test by opening the function URL in a web browser, appending?quantity=5&price=3
to the URL – you'll see{"total_cost":15}
in the browser.)
Integrating the Function into Copilot Studio as an Action
Our function is now deployed and accessible via HTTPS. The next step is to let our Copilot Studio AI agent use this function. In Copilot Studio, we can create a custom action that calls our function's API. Copilot actions allow the AI to do things like call external APIs or services when generating responses. We will set up a REST API action for the cost calculator.
Follow these steps to integrate the function into Copilot Studio:
- Open your agent's Actions in Copilot Studio: In Microsoft Copilot Studio, go to your AI Agent (or create a new custom agent). Navigate to the Actions tab for that agent, and click Add an action.
- Choose "REST API" as the action type: Copilot Studio provides a wizard to guide you. Select the option to add a REST API-based action (a custom connector). Copilot Studio actually makes this easy with a step-by-step wizard.
- Provide the API specification: The wizard will ask for an API specification (OpenAPI definition) for your function. Essentially, you need to describe your cost calculator endpoint in a format Copilot understands. For a simple function like ours, this spec can be very basic: one endpoint (the URL from DigitalOcean) with two parameters (
quantity
andprice
) and a JSON response containingtotal_cost
. (Note: Copilot Studio currently requires an OpenAPI v2 (Swagger) spec for custom API actions. If you have a v3 spec, the system will convert it, but it's easiest to write it as v2.) Describe the endpoint as an HTTP GET (or POST) that returns a response with the total cost. - Skip authentication (if not needed): Our DigitalOcean Function endpoint is public (it has a unique URL that security through obscurity). That means we don't need API keys or OAuth for this example. In the Copilot wizard, you can choose "No auth" or skip any auth configuration.
- Define inputs and output: As you continue through the wizard, you'll define how Copilot Studio should handle inputs/outputs. Map the
quantity
andprice
parameters from your API spec to the action's inputs (so the Copilot agent knows it needs those values to call the action). Then note the output field (total_cost
) from the response. Copilot Studio will use this to capture the result when the action is called. - Complete and publish the action: Finish the wizard, giving your action a name like "Cost Calculator". Save/publish the action for your agent. Once published, it may require an administrator to approve or enable it for use (depending on your organisation's settings). After it's approved, the action becomes available to your Copilot agent.
- Use the action in conversation: Now comes the fun part – using the action. You can explicitly call the action in one of your conversation Topics. For example, create a topic that triggers when the user asks about price or cost, then add a node to Invoke an action and choose the "Cost Calculator" action. The agent will prompt for the needed inputs (if not already provided by the user's question) and then call the function. Once the function returns the total cost, you can have the bot reply with something like, "The total cost is $X".
If you're using the Copilot generative AI mode, the agent might even choose to call this action on its own when it sees a relevant user question. That means if a user asks a price question, the AI can identify that the Cost Calculator action is needed and execute it, then blend the result into its answer – all automatically.
Conclusion
We’ve now created a simple but powerful enhancement for our AI chatbot. By leveraging DigitalOcean Functions, we wrote a few lines of Python to handle a specific task (calculating a price quote) and deployed it with minimal effort. DigitalOcean’s serverless platform handled the hosting and scaling for us, so we could focus on logic. As a result, our Copilot Studio agent gained a new capability without complicating its core dialogue flow.
This approach is beginner-friendly yet scalable. You can extend it to many other use-cases: from fetching live data (weather, stock prices) to performing database operations or sending notifications – all via small functions that your AI agent can call. DigitalOcean Functions offers the flexibility and ease to spin up these mini-APIs quickly
DigitalOcean and Copilot Studio makes it straightforward to incorporate them as actions.
By keeping your chatbots' brain (the AI model) separate from these utility functions, you also make your system more maintainable. You can update the function independently or reuse it across different bots or platforms.