Converting an SVG to PNG using PowerShell
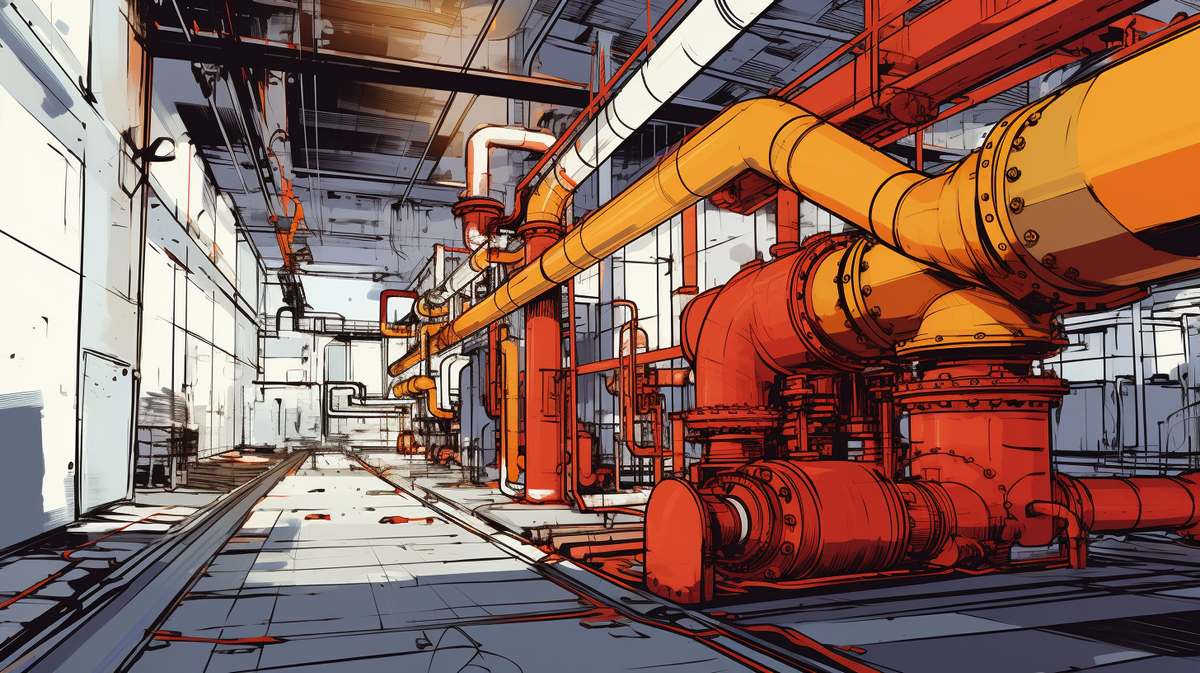
As part of another project I am working on, I was messing around with a tool called Gephi, which creates graphs from data. You give it a bunch of nodes and edges to model how each node relates to the next... think of it like a massive social network of friends and how they are all related to one another based on connections.
One of the challenges I faced with this project was when using large datasets of 100K+ nodes, the process of exporting and opening an SVG with that many objects is a little taxing even for the best image editing software.
In the end, I decided to write a small PowerShell script that would import the SVG, and using Inkscapes exe, you can convert this into a PNG. Pretty awesome.
The PowerShell script
The script's premise is to import the SVG, calculate the dimensions/aspect ratio of the vector image, and use this to output a correctly sized bitmap representation. Simple.
param(
[string]$sourceSvg = 'E:\Gephi\Map.svg',
[string]$inkscapeExec = 'C:\Program Files\Inkscape\bin\inkscape.exe',
[double]$scale = 1.0
)
# Check if source SVG file exists
if (-not (Test-Path $sourceSvg)) {
Write-Host "File $sourceSvg not found."
exit
}
# Extracting SVG dimensions
[xml]$svgContent = Get-Content -Path $sourceSvg
$width = [double]($svgContent.svg.width -replace "px", "")
$height = [double]($svgContent.svg.height -replace "px", "")
# Scaling dimensions
$scaledWidth = [int]($width * $scale)
$scaledHeight = [int]($height * $scale)
# Define target PNG name
$targetPng = [System.IO.Path]::ChangeExtension($sourceSvg, 'png')
Write-Host "Converting $sourceSvg (W: $width, H: $height) to $targetPng with scaled dimensions W: $scaledWidth, H: $scaledHeight..."
$command = "& `"$inkscapeExec`" --export-filename=`"$targetPng`" -w $scaledWidth -h $scaledHeight `"$sourceSvg`""
Invoke-Expression $command
I hope you find this useful the next time you have an SVG so large it can't be opened... 🤣
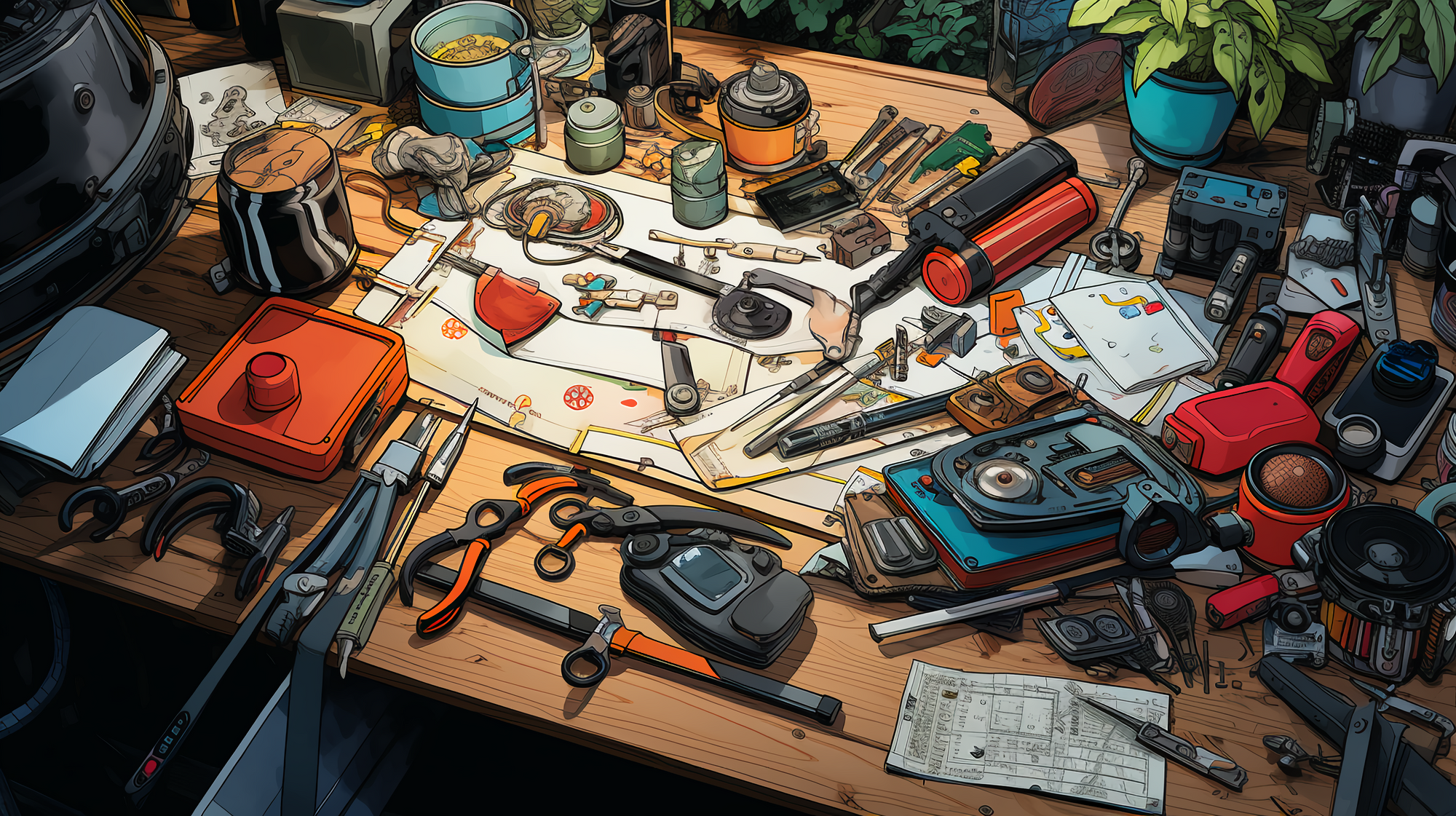
This is part of the Toolbox Tuesday series
A range of tools and tricks for your tech toolbox, from PowerShell scripts to document templates!
View more articles